A function is a block of code which only runs when it is called.
You can pass data, known as parameters, into a function.
Functions are used to perform certain actions, and they are important for reusing code: Define the code once, and use it many times.
Function declaration is required when you define a function in one source file and you call that function in another file. In such case, you should declare the function at the top of the file calling the function. Calling a Function. While creating a C function, you give a definition of what the function has to do. Now you've got the function setup (either before the main function, or after with a function prototype before) - you can go ahead and call it from the main function using the process we talked about earlier (while we're talking about this - it's worth noting that functions can call each other if you want this nested-like functionality with multiple functions).
Create a Function
C++ provides some pre-defined functions, such as main()
, which is used to execute code. But you can also create your own functions to perform certain actions.
To create (often referred to as declare) a function, specify the name of the function, followed by parentheses ():
Syntax
Example Explained
myFunction()
is the name of the functionvoid
means that the function does not have a return value. You will learn more about return values later in the next chapter- inside the function (the body), add code that defines what the function should do
Call a Function
Declared functions are not executed immediately. They are 'saved for later use', and will be executed later, when they are called.
To call a function, write the function's name followed by two parentheses ()
and a semicolon ;
In the following example, myFunction()
is used to print a text (the action), when it is called:
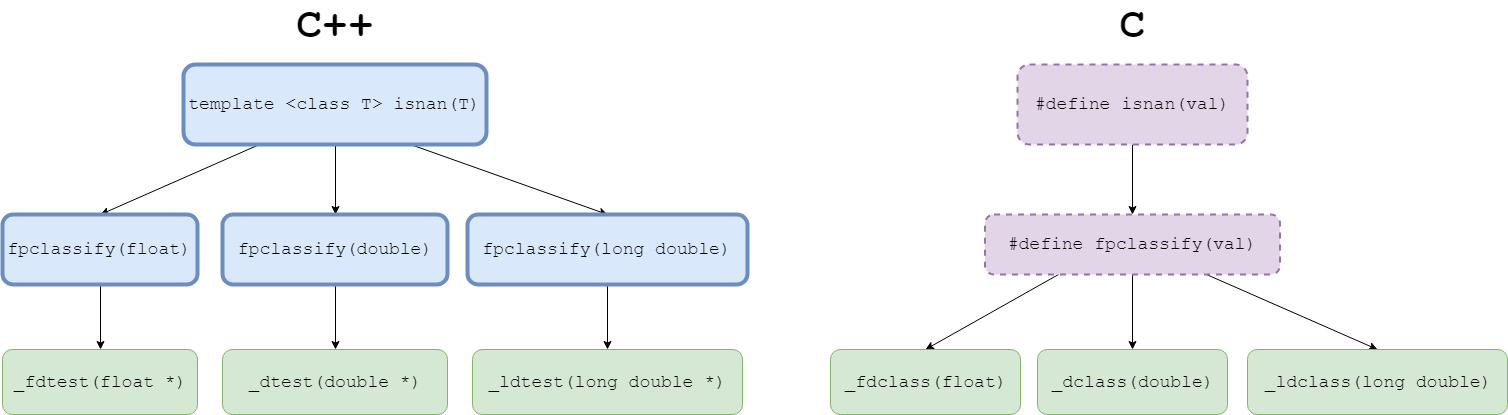
Example
Inside main
, call myFunction()
:
void myFunction() {
cout << 'I just got executed!';
}
int main() {
myFunction(); // call the function
return 0;
}
// Outputs 'I just got executed!'
A function can be called multiple times:
Example
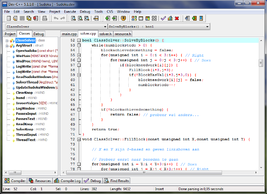
cout << 'I just got executed!n';
}
int main() {
myFunction();
myFunction();
myFunction();
return 0;
}
// I just got executed!
// I just got executed!
// I just got executed!
Function Declaration and Definition
A C++ function consist of two parts:
- Declaration: the function's name, return type, and parameters (if any)
- Definition: the body of the function (code to be executed)
// the body of the function (definition)
}
Note: If a user-defined function, such as myFunction()
is declared after the main()
function, an error will occur. It is because C++ works from top to bottom; which means that if the function is not declared above main()
, the program is unaware of it:
Example
myFunction();
return 0;
}
void myFunction() {
cout << 'I just got executed!';
}
// Error
However, it is possible to separate the declaration and the definition of the function - for code optimization.
You will often see C++ programs that have function declaration above main()
, and function definition below main()
. This will make the code better organized and easier to read:
Example
void myFunction();
// The main method
int main() {
myFunction(); // call the function
return 0;
}
// Function definition
void myFunction() {
cout << 'I just got executed!';
}